- This tool will help to install and the libraries and other tools to support react development.Let’s start with nodejs installation post completion on nodejs we will install create-react-app command line and will create a new react project1.Download nodejsVisit nodejs download page hereClick on macOS Installer to download the latest version of.
- The below packages include everything needed to get started with AppJS, including Node.js, all dependencies, binaries, and a launcher ready to go out of the box. 1.) Extract to a folder. 2.) Double click on launch. 3.) Hello World. AppJS 0.0.20 Distributables: Linux 32 bit / 64 bit- app.sh; Mac- app.sh; Windows- app.exe.
How to Run a Node.js Application on a Mac. Webucator provides instructor-led training to students throughout the US and Canada. We have trained over 90,000 students from over 16,000 organizations on technologies such as Microsoft ASP.NET, Microsoft Office, Azure, Windows, Java, Adobe, Python, SQL, JavaScript, Angular and much more. PS, there is not (yet) a node.js equivalent to py2app, etc., that would make the intermediate solution really easy. From what I understand, appjs.org is the most promising option at this point, but the current release doesn't work on Mac so you'll still have to do a good deal of work even if you go with the theoretically 'easy' way.
- Node.js Tutorial
- Node.js Useful Resources
- Selected Reading
Before creating an actual 'Hello, World!' application using Node.js, let us see the components of a Node.js application. A Node.js application consists of the following three important components −
Import required modules − We use the require directive to load Node.js modules.
Create server − A server which will listen to client's requests similar to Apache HTTP Server.
Read request and return response − The server created in an earlier step will read the HTTP request made by the client which can be a browser or a console and return the response.
Creating Node.js Application
Step 1 - Import Required Module
We use the require directive to load the http module and store the returned HTTP instance into an http variable as follows −
Uninstall Node Js On Mac
Step 2 - Create Server
We use the created http instance and call http.createServer() method to create a server instance and then we bind it at port 8081 using the listen method associated with the server instance. Pass it a function with parameters request and response. Write the sample implementation to always return 'Hello World'.
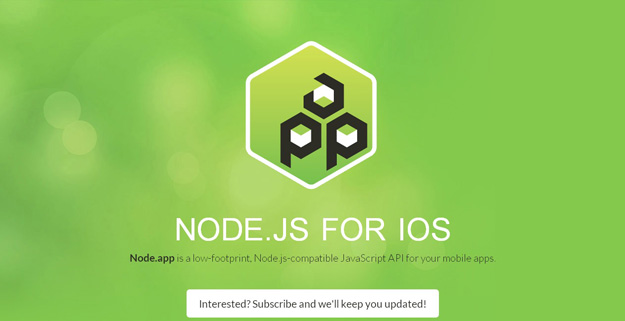
The above code is enough to create an HTTP server which listens, i.e., waits for a request over 8081 port on the local machine.
Step 3 - Testing Request & Response
Let's put step 1 and 2 together in a file called main.js and start our HTTP server as shown below −
Node Js Mac Application
Now execute the main.js to start the server as follows −
Verify the Output. Server has started.
Make a Request to the Node.js Server
Open http://127.0.0.1:8081/ in any browser and observe the following result.
Congratulations, you have your first HTTP server up and running which is responding to all the HTTP requests at port 8081.
In this tutorial, I’ll be teaching you how you can install Node.js on windows, mac or linux in under 15 minutes.
Node is an open-source runtime environment for javascript. It is a cross-platform environment providing its support for Mac, Windows and Linux. It runs Chrome’s V8 javascript engine, outside the browser, and because of this, it is very powerful.
It runs in a single process, without creating a new thread for every request. Node JS
How Does a Node App Look?
curl-sL https://deb.nodesource.com/setup_10.x | sudo -E bash - sudo apt-get install -y nodejs |
Once you download Node, the NPM or Node Package Manager automatically gets installed as it also ships with the node.
Installing the Text Editor
The next thing is to install a text editor which will help you in writing code. The one which I personally use is Visual Studio Code by Microsoft.
You can download it from here: https://code.visualstudio.com/
Once you are done with the text editor, the next thing which you can do is to check whether the path of the Node is correct or not.
Testing Nodejs and NPM installation
You can check your node installation just by running a small node command which will show you the version installed. Just open your cmd or PowerShell and paste the below command. This will show the version of node installed on your computer.
2 | v12.16.0 |
Similarly, you can check the version of npm installed on your computer. Just paste the below node command to check the version.
2 | v6.13.4 |
Running Your First App
Here you are all set up, let’s try to run a small node server. Open VS Code and create a new file and save it as app.js
Now paste the following code.
2 | Server running at http://127.0.0.1:3000/ |
You can now visit http://127.0.0.1:3000/
to check your node app running.
Well, this was all about setting up your system for js development. If you stuck into any kind of error, don’t forget to google it and try to debug it on your own.
This will teach you how to debug on your own as someone might have faced a similar problem earlier.
If you still don’t find any solution for your problem, you can ask your doubt in the comment’s section below and we’ll get back to you.